I just make a script to check dependencies
It check all dependencies in a directory, that should come in handy when you're compiling

At the same time, it shows which libraries are absent

The result goes to standard output when the absent one goes to standard error
There're 4 different types of output
With option -f, you'll get a full list of sorted dependencies
with option -l, you'll get a long version of tcz list
with option -s, you'll get a short version of tcz list (similar to chkonboot.sh)
with option -n, you'll get a list of libraries that were not found (it goes to standard error)
The idea is quite simple
1. find all files and run ldd to check all dependencies
2. generate a full list
3. remove the files that are under the directory from the full list
4. generate a "NOTFOUND" list
5. generate a full tcz list
6. remove all redundant
Usage: get-dep-list [-option] your_top_directory
Available Options (Default -ns)
-f show full dependencies list
-h show help
-l show detected tczs (long)
-n show dependencies that are not found
-s show detected tczs (short)
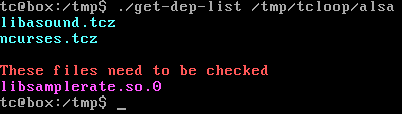
Tested on 7.2 x86
Boot base norestore
tce-load only alsa
As you can see, the libsamplerate.so.0 is missing
After loading the libsamplerate.tcz extension fix the problem
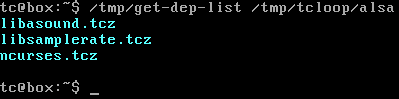
By the way ~
Please add as a dependency, thanks.

However, the KERNEL module were not listed, extensions like these should be handled with care
Code here:
#!/bin/sh
# author polikuo
# version 1.0
# Dec 4 2016
. /etc/init.d/tc-functions
useBusybox
showhelp() {
echo ${YELLOW}
echo 'Usage: '"$(basename $0)" [-option] your_top_directory
echo ' 'Available Options \(Default -ns\)
echo ' '\-f show full dependencies list
echo ' '\-h show help
echo ' '\-l show detected tczs \(long\)
echo ' '\-n show dependencies that are not found
echo ' '\-s show detected tczs \(short\)
echo ${NORMAL}
exit 1
}
unset TCEDIR LIBS NOTFOUND ELF TCZS file DEP DELETE not_needed LIST NN NF option B D F L O N S T DIR SELF REMOVE
DIR=false
for O in $@;
do
echo $O | grep -Eqv "^-" && {
cd $O 2> /dev/null && { DIR=true; break; } || { echo ${RED}can\'t cd to $O${NORMAL}; break; }
}
done
(( $DIR )) || showhelp
D=0
F=false
L=false
N=false
S=false
while getopts fhlns option
do
case "$option" in
f ) F=true; D=$((D+1));;
h ) showhelp;;
l ) L=true; D=$((D+1));;
n ) N=true; D=$((D+1));;
s ) S=true; D=$((D+1));;
* ) showhelp;;
esac
done
[ $D -eq 0 ] && { N=true;S=true; }
# Preparing
TCEDIR=/etc/sysconfig/tcedir
ELF="$(find . -not -type d -exec ldd {} + 2> /dev/null | cut -d '(' -f 1 | tr -d '\t' | sort | uniq)"
SELF="$(for B in `find . -not -type d`; do basename $B; done | grep -Ev "\.$")"
LIBS="$(echo "$ELF" | tr -d ' ' | grep -v "notfound" | cut -d '>' -f 2)"
for REMOVE in $SELF
do
LIBS="$(echo "$LIBS" | grep -v $REMOVE)"
done
NOTFOUND="$(echo "$ELF" | tr -d ' ' | grep "notfound" | cut -d '=' -f 1)"
TCZS="$(ls -l $LIBS 2> /dev/null | grep /tmp/tcloop/ | cut -d '>' -f 2 | cut -d '/' -f 4 | sort | uniq | sed 's/$/\.tcz/g')"
(( $F )) && echo "$ELF" && echo 1>&0
(( $L )) && echo "$TCZS" && echo 1>&0
# checking if TCZS existed
for file in $TCZS
do
[ -s "$TCEDIR"/optional/"$file" ] || {
echo ${MAGENTA}$file not found or size is zero${NORMAL}
echo If you load it elsewhere, please move it and the dep file into your
echo ${CYAN}$(ls -l /etc/sysconfig | grep tcedir | awk '{ print $11 "optional/" }')${NORMAL}
exit 1
}
done
# checking for repeated
chkrpt() {
for DEP in $TCZS
do
if [ -s "$TCEDIR"/optional/"$DEP".dep ]
then
for DELETE in $(cat "$TCEDIR"/optional/"$DEP".dep)
do
if ( echo "$TCZS" | grep -q "$DELETE" )
then
not_needed="$not_needed"" $DELETE"
fi
done
fi
done
LIST="$TCZS"
for NN in `echo $not_needed | tr ' ' '\n' | sort | uniq`
do
LIST=`echo $LIST | sed "s/$NN//g"`
done
for DEP in $LIST
do
echo ${CYAN}$DEP${NORMAL}
done
}
(( $S )) && chkrpt && echo 1>&0
# Show NOTFOUND
snf() {
[ "$(echo "$NOTFOUND" | tr -d ' ')" = '' ] || {
echo ${RED}"These files need to be checked"${NORMAL} 1>&2
for NF in $NOTFOUND; do
echo ${MAGENTA}$NF${NORMAL}
done | sort | uniq 1>&2
}
}
(( $N )) && snf
I've found many extensions either having missing dependencies or loading too much extensions
I'm afraid I can't list them all, but I hope this script would help
Feedbacks are welcomed
